[Pkg-javascript-commits] [node-balanced-match] 01/02: Imported Upstream version 0.2.0
Sebastiaan Couwenberg
sebastic at moszumanska.debian.org
Tue Mar 17 13:39:51 UTC 2015
This is an automated email from the git hooks/post-receive script.
sebastic pushed a commit to branch master
in repository node-balanced-match.
commit db0ff4578a098f60d4395a518dcb3259670d5967
Author: Bas Couwenberg <sebastic at xs4all.nl>
Date: Tue Mar 17 13:51:22 2015 +0100
Imported Upstream version 0.2.0
---
.gitignore | 2 ++
.travis.yml | 4 +++
Makefile | 6 +++++
README.md | 80 ++++++++++++++++++++++++++++++++++++++++++++++++++++++++
example.js | 5 ++++
index.js | 38 +++++++++++++++++++++++++++
package.json | 47 +++++++++++++++++++++++++++++++++
test/balanced.js | 56 +++++++++++++++++++++++++++++++++++++++
8 files changed, 238 insertions(+)
diff --git a/.gitignore b/.gitignore
new file mode 100644
index 0000000..fd4f2b0
--- /dev/null
+++ b/.gitignore
@@ -0,0 +1,2 @@
+node_modules
+.DS_Store
diff --git a/.travis.yml b/.travis.yml
new file mode 100644
index 0000000..cc4dba2
--- /dev/null
+++ b/.travis.yml
@@ -0,0 +1,4 @@
+language: node_js
+node_js:
+ - "0.8"
+ - "0.10"
diff --git a/Makefile b/Makefile
new file mode 100644
index 0000000..fa5da71
--- /dev/null
+++ b/Makefile
@@ -0,0 +1,6 @@
+
+test:
+ @node_modules/.bin/tape test/*.js
+
+.PHONY: test
+
diff --git a/README.md b/README.md
new file mode 100644
index 0000000..2aff0eb
--- /dev/null
+++ b/README.md
@@ -0,0 +1,80 @@
+# balanced-match
+
+Match balanced string pairs, like `{` and `}` or `<b>` and `</b>`.
+
+[](http://travis-ci.org/juliangruber/balanced-match)
+[](https://www.npmjs.org/package/balanced-match)
+
+[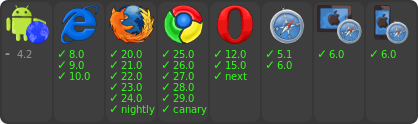](https://ci.testling.com/juliangruber/balanced-match)
+
+## Example
+
+Get the first matching pair of braces:
+
+```js
+var balanced = require('balanced-match');
+
+console.log(balanced('{', '}', 'pre{in{nested}}post'));
+console.log(balanced('{', '}', 'pre{first}between{second}post'));
+```
+
+The matches are:
+
+```bash
+$ node example.js
+{ start: 3, end: 14, pre: 'pre', body: 'in{nested}', post: 'post' }
+{ start: 3,
+ end: 9,
+ pre: 'pre',
+ body: 'first',
+ post: 'between{second}post' }
+```
+
+## API
+
+### var m = balanced(a, b, str)
+
+For the first non-nested matching pair of `a` and `b` in `str`, return an
+object with those keys:
+
+* **start** the index of the first match of `a`
+* **end** the index of the matching `b`
+* **pre** the preamble, `a` and `b` not included
+* **body** the match, `a` and `b` not included
+* **post** the postscript, `a` and `b` not included
+
+If there's no match, `undefined` will be returned.
+
+If the `str` contains more `a` than `b` / there are unmatched pairs, the first match that was closed will be used. For example, `{{a}` will match `['{', 'a', '']`.
+
+## Installation
+
+With [npm](https://npmjs.org) do:
+
+```bash
+npm install balanced-match
+```
+
+## License
+
+(MIT)
+
+Copyright (c) 2013 Julian Gruber <julian at juliangruber.com>
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
+of the Software, and to permit persons to whom the Software is furnished to do
+so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/example.js b/example.js
new file mode 100644
index 0000000..c02ad34
--- /dev/null
+++ b/example.js
@@ -0,0 +1,5 @@
+var balanced = require('./');
+
+console.log(balanced('{', '}', 'pre{in{nested}}post'));
+console.log(balanced('{', '}', 'pre{first}between{second}post'));
+
diff --git a/index.js b/index.js
new file mode 100644
index 0000000..d165ae8
--- /dev/null
+++ b/index.js
@@ -0,0 +1,38 @@
+module.exports = balanced;
+function balanced(a, b, str) {
+ var bal = 0;
+ var m = {};
+ var ended = false;
+
+ for (var i = 0; i < str.length; i++) {
+ if (a == str.substr(i, a.length)) {
+ if (!('start' in m)) m.start = i;
+ bal++;
+ }
+ else if (b == str.substr(i, b.length) && 'start' in m) {
+ ended = true;
+ bal--;
+ if (!bal) {
+ m.end = i;
+ m.pre = str.substr(0, m.start);
+ m.body = (m.end - m.start > 1)
+ ? str.substring(m.start + a.length, m.end)
+ : '';
+ m.post = str.slice(m.end + b.length);
+ return m;
+ }
+ }
+ }
+
+ // if we opened more than we closed, find the one we closed
+ if (bal && ended) {
+ var start = m.start + a.length;
+ m = balanced(a, b, str.substr(start));
+ if (m) {
+ m.start += start;
+ m.end += start;
+ m.pre = str.slice(0, start) + m.pre;
+ }
+ return m;
+ }
+}
diff --git a/package.json b/package.json
new file mode 100644
index 0000000..0181423
--- /dev/null
+++ b/package.json
@@ -0,0 +1,47 @@
+{
+ "name": "balanced-match",
+ "description": "Match balanced character pairs, like \"{\" and \"}\"",
+ "version": "0.2.0",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/juliangruber/balanced-match.git"
+ },
+ "homepage": "https://github.com/juliangruber/balanced-match",
+ "main": "index.js",
+ "scripts": {
+ "test": "make test"
+ },
+ "dependencies": {},
+ "devDependencies": {
+ "tape": "~1.1.1"
+ },
+ "keywords": [
+ "match",
+ "regexp",
+ "test",
+ "balanced",
+ "parse"
+ ],
+ "author": {
+ "name": "Julian Gruber",
+ "email": "mail at juliangruber.com",
+ "url": "http://juliangruber.com"
+ },
+ "license": "MIT",
+ "testling": {
+ "files": "test/*.js",
+ "browsers": [
+ "ie/8..latest",
+ "firefox/20..latest",
+ "firefox/nightly",
+ "chrome/25..latest",
+ "chrome/canary",
+ "opera/12..latest",
+ "opera/next",
+ "safari/5.1..latest",
+ "ipad/6.0..latest",
+ "iphone/6.0..latest",
+ "android-browser/4.2..latest"
+ ]
+ }
+}
diff --git a/test/balanced.js b/test/balanced.js
new file mode 100644
index 0000000..36bfd39
--- /dev/null
+++ b/test/balanced.js
@@ -0,0 +1,56 @@
+var test = require('tape');
+var balanced = require('..');
+
+test('balanced', function(t) {
+ t.deepEqual(balanced('{', '}', 'pre{in{nest}}post'), {
+ start: 3,
+ end: 12,
+ pre: 'pre',
+ body: 'in{nest}',
+ post: 'post'
+ });
+ t.deepEqual(balanced('{', '}', '{{{{{{{{{in}post'), {
+ start: 8,
+ end: 11,
+ pre: '{{{{{{{{',
+ body: 'in',
+ post: 'post'
+ });
+ t.deepEqual(balanced('{', '}', 'pre{body{in}post'), {
+ start: 8,
+ end: 11,
+ pre: 'pre{body',
+ body: 'in',
+ post: 'post'
+ });
+ t.deepEqual(balanced('{', '}', 'pre}{in{nest}}post'), {
+ start: 4,
+ end: 13,
+ pre: 'pre}',
+ body: 'in{nest}',
+ post: 'post'
+ });
+ t.deepEqual(balanced('{', '}', 'pre{body}between{body2}post'), {
+ start: 3,
+ end: 8,
+ pre: 'pre',
+ body: 'body',
+ post: 'between{body2}post'
+ });
+ t.notOk(balanced('{', '}', 'nope'), 'should be notOk');
+ t.deepEqual(balanced('<b>', '</b>', 'pre<b>in<b>nest</b></b>post'), {
+ start: 3,
+ end: 19,
+ pre: 'pre',
+ body: 'in<b>nest</b>',
+ post: 'post'
+ });
+ t.deepEqual(balanced('<b>', '</b>', 'pre</b><b>in<b>nest</b></b>post'), {
+ start: 7,
+ end: 23,
+ pre: 'pre</b>',
+ body: 'in<b>nest</b>',
+ post: 'post'
+ });
+ t.end();
+});
--
Alioth's /usr/local/bin/git-commit-notice on /srv/git.debian.org/git/pkg-javascript/node-balanced-match.git
More information about the Pkg-javascript-commits
mailing list